Simplifying Unix Epoch Timestamps in PowerShell Using DateTimeOffset
Handling Unix Epoch timestamps in PowerShell can be cumbersome, but with DateTimeOffset, you can streamline conversions with minimal effort.
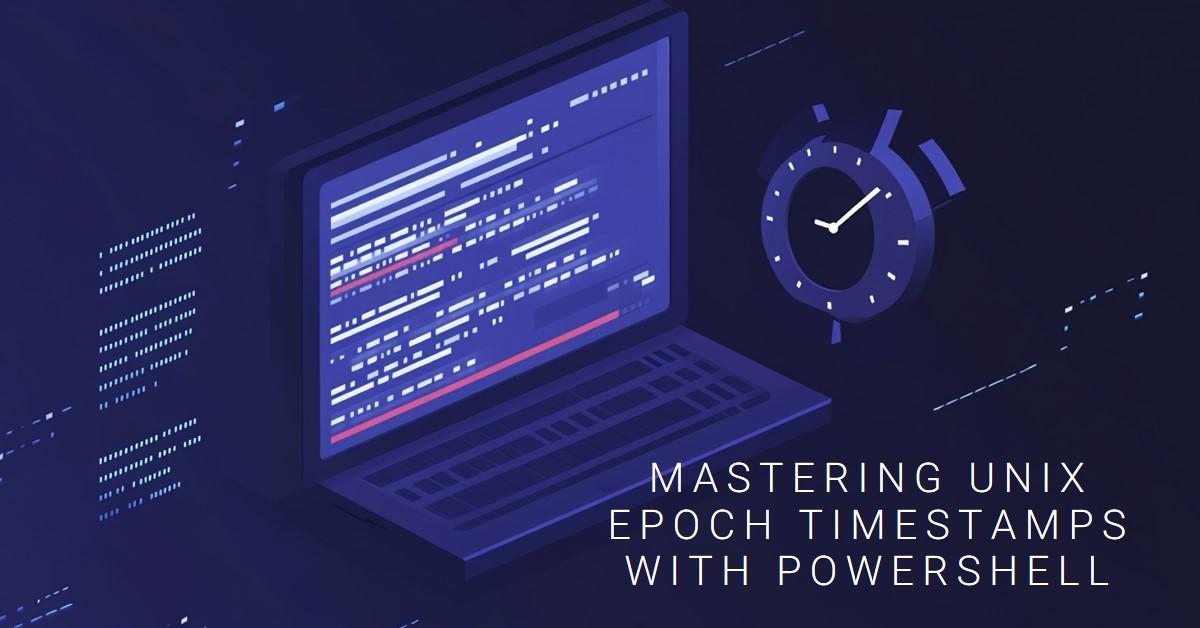
If you’ve ever dealt with Unix Epoch time in PowerShell, you know it can be a hassle. By default, Get-Date
doesn’t output or accept Epoch time, forcing users to manually calculate the seconds between 1970 and their target date.
PS C:\> # Examples of Previous ways to calculate current Unix Time
PS C:\> [int][double]::Parse((Get-Date -UFormat %s))
1724142672
PS C:\> [System.Math]::Truncate((Get-Date -UFormat %s))
1724142720
Fortunately, there’s a better way. The .NET DateTimeOffset
class provides an efficient method to handle Unix Epoch timestamps directly in PowerShell.
PS C:\> $Date = Get-Date
PS C:\> [System.DateTimeOffset]::new($Date)
DateTime : 20/08/2024 7:54:59 PM
UtcDateTime : 20/08/2024 7:54:59 AM
LocalDateTime : 20/08/2024 7:54:59 PM
Date : 20/08/2024 12:00:00 AM
Day : 20
DayOfWeek : Tuesday
DayOfYear : 233
Hour : 19
Millisecond : 287
Microsecond : 557
Nanosecond : 0
Minute : 54
Month : 8
Offset : 12:00:00
TotalOffsetMinutes : 720
Second : 59
Ticks : 638597804992875570
UtcTicks : 638597372992875570
TimeOfDay : 19:54:59.2875570
Year : 2024
While the output doesn’t display Unix Time by default, the DateTimeOffset object includes methods to convert to Unix Time in either seconds or milliseconds, depending on your accuracy needs.
For example, to get the current time in Unix Epoch seconds:
PS C:\> [System.DateTimeOffset]::new( (Get-Date) ).ToUnixTimeSeconds()
1724140499
Get-Date
can be swapped out for any other PowerShell DateTime object to convert an existing timestamp.
Converting from an existing Unix timestamp is even easier. The DateTimeOffset
class has a FromUnixTimeSeconds
method, allowing you to convert back to a human-readable date and time object.
For instance, converting the Unix time from the previous example back to a readable format:
PS C:\> $UnixTimestamp = "1724140499"
PS C:\> [DateTimeOffset]::FromUnixTimeSeconds($UnixTimestamp)
DateTime : 20/08/2024 7:54:59 AM
UtcDateTime : 20/08/2024 7:54:59 AM
LocalDateTime : 20/08/2024 7:54:59 PM
Date : 20/08/2024 12:00:00 AM
Day : 20
DayOfWeek : Tuesday
DayOfYear : 233
Hour : 7
Millisecond : 0
Microsecond : 0
Nanosecond : 0
Minute : 54
Month : 8
Offset : 00:00:00
TotalOffsetMinutes : 0
Second : 59
Ticks : 638597372990000000
UtcTicks : 638597372990000000
TimeOfDay : 07:54:59
Year : 2024
Short and to the point, but I hope this helps next time you’re working with Unix Epoch timestamps in PowerShell!